Cross-Site Scripting Explained: Protect Your Website from Modern Threats

Cross-Site Scripting (XSS) is a type of security vulnerability that allows attackers to inject malicious scripts into websites. These scripts are often executed in the web browser of users who visit the site, leading to unintended and potentially harmful actions. XSS is primarily used to steal user data, hijack user sessions, deface websites, or spread malware.
How Does XSS Work?
Imagine a website that allows users to submit comments on a blog post. If the site does not properly sanitize the input, an attacker could inject a script instead of a harmless comment. When another user views the comment, the browser executes the script as if it were part of the website, leading to various harmful outcomes.

Types of XSS
1. Stored XSS: Malicious scripts are stored on the target server (e.g., in a database) and are executed when users retrieve the data. This is particularly dangerous because the attack can affect many users.
2. Reflected XSS: The malicious script is reflected off a web server, such as in a search query or an error message, and executed immediately in the user’s browser.
3. DOM-based XSS: The vulnerability exists in the client-side code rather than the server-side, often through manipulating the Document Object Model (DOM) environment in the user’s browser.
Why Should You Care About XSS?
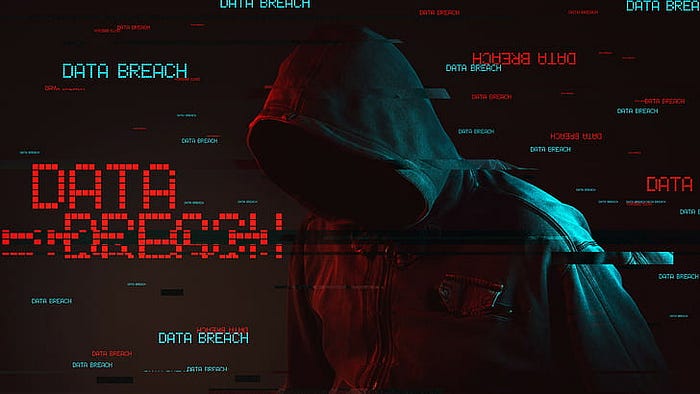
XSS is not just a technical issue — it’s a business problem. Here’s why:
1. Data Breaches: Attackers can steal sensitive data, including user credentials, personal information, or payment details, leading to severe privacy violations.
2. Brand Reputation: A successful XSS attack can damage your brand’s reputation. Users losing trust in your website can lead to a significant drop in traffic and revenue.
3. Legal Consequences: Failure to protect user data can result in legal actions, especially with regulations like GDPR in effect.
4. Financial Losses: Beyond fines and legal costs, a drop in user confidence can directly impact sales and profitability.
Is XSS Still a Threat in 2024?
Yes, XSS remains a threat in 2024. While developers are more aware of XSS vulnerabilities, the web landscape continues to evolve, introducing new frameworks and technologies that can inadvertently open the door to XSS attacks if not properly handled.
Moreover, the increasing use of client-side scripting and complex single-page applications (SPAs) provides new vectors for XSS attacks. As attackers become more sophisticated, the need for robust security practices is more critical than ever.
How to Avoid XSS Vulnerabilities

Preventing XSS involves a combination of secure coding practices, proper input validation, and output encoding. Here’s how you can protect your website:
1. Input Validation and Sanitization
Always validate and sanitize user input. Reject or escape any input that contains potentially dangerous characters. For example:
```javascript
function sanitizeInput(input) {
return input.replace(/</g, "<").replace(/>/g, ">");
}
// Example of using sanitized input in HTML
document.getElementById('comment').innerHTML = sanitizeInput(userComment);
```
2. Use Secure APIs
When using APIs, prefer those that automatically escape data. For example, in modern frameworks like React, data binding automatically escapes any special characters, reducing the risk of XSS.
```javascript
// React automatically escapes special characters
const Comment = ({ text }) => <p>{text}</p>;
```
3. Content Security Policy (CSP)
Implement a Content Security Policy to restrict the sources from which scripts can be executed. CSP can mitigate the risk of XSS by blocking unauthorized scripts.
```html
<! - Example CSP header →
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' https://trusted.cdn.com">
```
4. Avoid Inline JavaScript
Avoid embedding JavaScript directly in HTML, such as in `onclick` attributes. Instead, use event listeners in external or internal JavaScript files.
```javascript
// Instead of this:
<button onclick="alert('Clicked!')">Click me</button>
// Use this:
document.getElementById('myButton').addEventListener('click', function() {
alert('Clicked!');
});
```
5. Escape Output
Always escape output when displaying user-generated content. This ensures that any embedded scripts are rendered harmless.
```php
// PHP example using htmlspecialchars
echo htmlspecialchars($userComment, ENT_QUOTES, 'UTF-8');
```
6. Regular Security Audits
Perform regular security audits and code reviews to catch vulnerabilities early. Automated tools can help, but manual reviews are also crucial for identifying complex issues.
Conclusion
Cross-Site Scripting (XSS) is a persistent threat that can have severe implications for your website and business. Despite advancements in web security, XSS remains relevant due to the evolving nature of web applications and attack techniques. By adopting secure coding practices, using modern frameworks, and staying vigilant with regular security checks, you can significantly reduce the risk of XSS and protect both your users and your business.
Remember, security is an ongoing process, not a one-time fix. Keep your knowledge up-to-date, stay aware of new vulnerabilities, and continue to refine your security practices. By doing so, you can create a safer web environment for everyone.